E2E tutorial
Contents
Get Actual CRC value from a CAN message
# example for getting actual CRC value from a CAN message with Profile 2
from project import *
from andi.e2e import get_actual_crc
# replace database_path with a correct path
database = andi.load_database(database_path)
# replace frame_id with a correct value
frame = database.get_frame_by_id(frame_id)
can_message = message_builder.create_can_message()
data = tuple(bytearray.fromhex('53 01 01 02 03 04 05 06 07 08 09 01 02'\
'03 04 05 06 07 08 09 01 02 03 04 05 06'\
'07 08 09 01 02 03'))
can_message.data_base = database
can_message.frame = frame
can_message.payload = data
print("Actual CRC : " + str(get_actual_crc(can_message)))
Get Actual counter value from a CAN message
# example for getting actual counter value from a CAN message with Profile 2
from project import *
from andi.e2e import get_actual_counter
# replace database_path with a correct path
database = andi.load_database(database_path)
# replace frame_id with a correct value
frame = database.get_frame_by_id(frame_id)
can_message = message_builder.create_can_message()
data = tuple(bytearray.fromhex('53 01 01 02 03 04 05 06 07 08 09 01 02'\
'03 04 05 06 07 08 09 01 02 03 04 05 06'\
'07 08 09 01 02 03'))
can_message.data_base = database
can_message.frame = frame
can_message.payload = data
print("Actual counter : " + str(get_actual_counter(can_message)))
Get Expected CRC value from a CAN message
# example for getting expected CRC value from a CAN message with Profile 2
from project import *
from andi.e2e import get_expected_crc
# replace database_path with a correct path
database = andi.load_database(database_path)
# replace frame_id with a correct value
frame = database.get_frame_by_id(frame_id)
can_message = message_builder.create_can_message()
data = tuple(bytearray.fromhex('53 01 01 02 03 04 05 06 07 08 09 01 02'\
'03 04 05 06 07 08 09 01 02 03 04 05 06'\
'07 08 09 01 02 03'))
can_message.data_base = database
can_message.frame = frame
can_message.payload = data
print("Expected CRC : " + str(get_expected_crc(can_message)))
E2E with SOME/IP-TP
# example for E2E methods with SOME/IP-TP messages
from project import *
from andi.e2e import increment_counter, protect
from andi.someip import segmentize
# replace differents variables with correct values
someip_message = message_builder.create_someip_message(sender, receiver)
someip_message.data_base = andi.load_database(database_path, "ethernet")
someip_message.someip_header.service_identifier = service_identifier
someip_message.someip_header.method_identifier = method_identifier
someip_message.someip_header.client_id = client_id
someip_message.someip_header.session_id = session_id
someip_message.someip_header.protocol_version = protocol_version
someip_message.someip_header.interface_version = interface_version
someip_message.someip_header.message_type = MessageType.NOTIFICATION
someip_message.someip_header.return_code = ReturnCode.E_OK
someip_message.payload = tuple(bytearray.fromhex('00') * 2000)
# protect the message after setting the payload and the different parameters
# protect updates the Length, DataId and CRC based on the e2e information in the database
protect(someip_message)
# in case we need to segmentize the message, this has to be done after protecting it
someip_segments = segmentize(someip_message, 1392)
for segment in someip_segments:
segment.send()
# in case someip_message is going to be sent more than once,
# incremeting the counter is needed before sending the next message
increment_counter(someip_message)
E2E Cutomized Configuration
In this section, we will explain how to create a customized configuration to parse E2E data in ARXML databases. The configuration, shown below, allows a flexible parsing based on specific conventions and profiles used by OEMs.
"E2E": {
"OEM": "YourOEMName",
"Config": [
{
"profile": "ProfileName1",
"checksum_key_words": ["CRC", "Checksum"],
"counter_key_words": ["Counter"],
"data_id_key_word": "DataId",
"max_counter_value": 255,
"similar_autosar_profile": "CRC_Profile_Name",
"protocol": "CAN"
}
]
}
Explanation of Fields
OEM: Specifies the Original Equipment Manufacturer, allowing for OEM-specific parsing rules.
Config: Contains a list of configurations for different E2E profiles.
profile: The name of the profile being defined. This can be used to distinguish between various E2E configurations.
checksum_key_words: Contains a list of keywords used to identify the checksum signals in the ARXML data. This allows flexibility in naming conventions.
counter_key_words: Contains a list of the keywords used to identify counter signals, enabling the parser to locate these signals based on various possible names.
data_id_key_word: Specifies the keyword used to identify the Data ID in the ARXML file. This assists in locating the relevant data identifiers.
max_counter_value: Defines the maximum value for the counter. If not specified, it can be dynamically retrieved based on the profile.
similar_autosar_profile: Refers to a related AUTOSAR profile for CRC calculation routines, allowing for easier integration with existing methods.
protocol: Specifies the concerned communication protocol. Currently, only "CAN" is supported. This can be extended based on OEMs' requests.
Usage
This configuration file can be loaded in the Settings form and be taken as input by the parsing system to dynamically adjust how it retrieves and interprets E2E data from ARXML files. By using keywords, the parser can effectively find and process the relevant signals, accommodating the varying standards and conventions used by different OEMs or profiles.
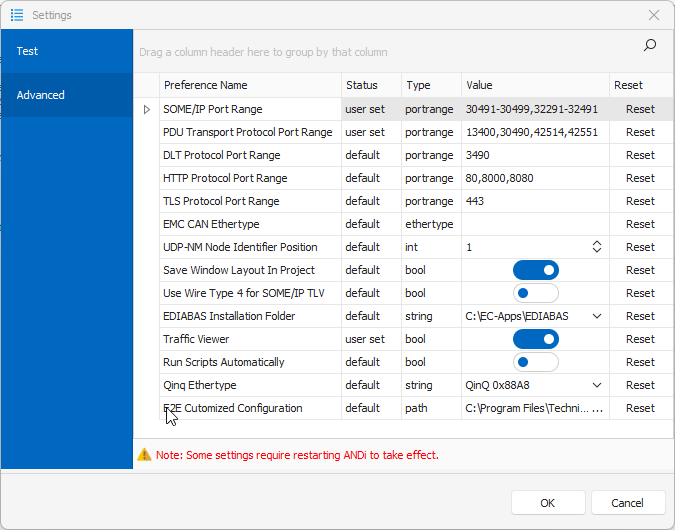